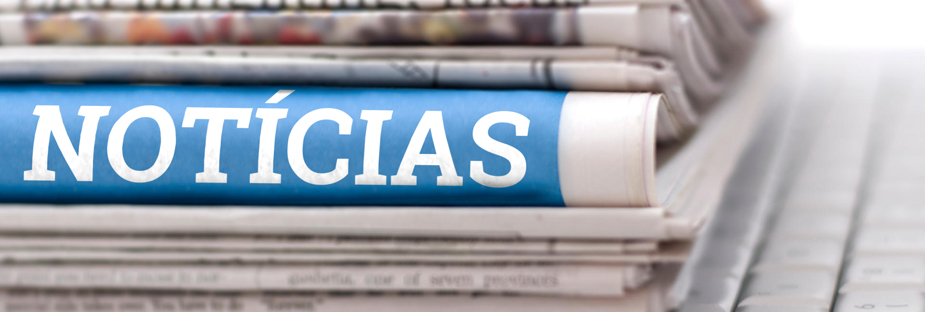
worst case complexity of insertion sort
worst case complexity of insertion sort
At each iteration, insertion sort removes one element from the input data, finds the location it belongs within the sorted list, and inserts it there. Algorithms are commonplace in the world of data science and machine learning. b) Quick Sort Lecture 18: INSERTION SORT in 1 Video [Theory + Code] || Best/Worst d) (1') The best case run time for insertion sort for a array of N . Please write comments if you find anything incorrect, or you want to share more information about the topic discussed above, An Insertion Sort time complexity question, C program for Time Complexity plot of Bubble, Insertion and Selection Sort using Gnuplot, Comparison among Bubble Sort, Selection Sort and Insertion Sort, Python Code for time Complexity plot of Heap Sort, Insertion sort to sort even and odd positioned elements in different orders, Count swaps required to sort an array using Insertion Sort, Difference between Insertion sort and Selection sort, Sorting by combining Insertion Sort and Merge Sort algorithms. For n elements in worst case : n*(log n + n) is order of n^2. Best and Worst Use Cases of Insertion Sort. The Big O notation is a function that is defined in terms of the input. The letter n often represents the size of the input to the function. Quicksort algorithms are favorable when working with arrays, but if data is presented as linked-list, then merge sort is more performant, especially in the case of a large dataset. Insertion Sort (With Code in Python/C++/Java/C) - Programiz However, a disadvantage of insertion sort over selection sort is that it requires more writes due to the fact that, on each iteration, inserting the (k+1)-st element into the sorted portion of the array requires many element swaps to shift all of the following elements, while only a single swap is required for each iteration of selection sort. Like selection sort, insertion sort loops over the indices of the array. The best case input is an array that is already sorted. Thus, swap 11 and 12. The outer loop runs over all the elements except the first one, because the single-element prefix A[0:1] is trivially sorted, so the invariant that the first i entries are sorted is true from the start. Data Scientists are better equipped to implement the insertion sort algorithm and explore other comparable sorting algorithms such as quicksort and bubble sort, and so on. a) (j > 0) || (arr[j 1] > value) What is not true about insertion sort?a. [1], D.L. In each step, the key under consideration is underlined. And it takes minimum time (Order of n) when elements are already sorted. What is Insertion Sort Algorithm: How it works, Advantages Move the greater elements one position up to make space for the swapped element. Best-case, and Amortized Time Complexity Worst-case running time This denotes the behaviour of an algorithm with respect to the worstpossible case of the input instance. STORY: Kolmogorov N^2 Conjecture Disproved, STORY: man who refused $1M for his discovery, List of 100+ Dynamic Programming Problems, Generating IP Addresses [Backtracking String problem], Longest Consecutive Subsequence [3 solutions], Cheatsheet for Selection Algorithms (selecting K-th largest element), Complexity analysis of Sieve of Eratosthenes, Time & Space Complexity of Tower of Hanoi Problem, Largest sub-array with equal number of 1 and 0, Advantages and Disadvantages of Huffman Coding, Time and Space Complexity of Selection Sort on Linked List, Time and Space Complexity of Merge Sort on Linked List, Time and Space Complexity of Insertion Sort on Linked List, Recurrence Tree Method for Time Complexity, Master theorem for Time Complexity analysis, Time and Space Complexity of Circular Linked List, Time and Space complexity of Binary Search Tree (BST), The worst case time complexity of Insertion sort is, The average case time complexity of Insertion sort is, If at every comparison, we could find a position in sorted array where the element can be inserted, then create space by shifting the elements to right and, Simple and easy to understand implementation, If the input list is sorted beforehand (partially) then insertions sort takes, Chosen over bubble sort and selection sort, although all have worst case time complexity as, Maintains relative order of the input data in case of two equal values (stable). sorting - Time Complexity of Insertion Sort - Stack Overflow ". One important thing here is that in spite of these parameters the efficiency of an algorithm also depends upon the nature and size of the input. I'm pretty sure this would decrease the number of comparisons, but I'm not exactly sure why. To order a list of elements in ascending order, the Insertion Sort algorithm requires the following operations: In the realm of computer science, Big O notation is a strategy for measuring algorithm complexity. Average-case analysis We wont get too technical with Big O notation here. It just calls insert on the elements at indices 1, 2, 3, \ldots, n-1 1,2,3,,n 1. The worst-case running time of an algorithm is . Expected Output: 1, 9, 10, 15, 30 Before going into the complexity analysis, we will go through the basic knowledge of Insertion Sort. Sanfoundry Global Education & Learning Series Data Structures & Algorithms. Direct link to Jayanth's post No sure why following cod, Posted 7 years ago. d) O(logn) Why is worst case for bubble sort N 2? Insertion Sort Interview Questions and Answers - Sanfoundry The Insertion Sort is an easy-to-implement, stable sort with time complexity of O(n2) in the average and worst case. T(n) = 2 + 4 + 6 + 8 + ---------- + 2(n-1), T(n) = 2 * ( 1 + 2 + 3 + 4 + -------- + (n-1)). a) Quick Sort It is known as the best sorting algorithm in Python. How do you get out of a corner when plotting yourself into a corner, Movie with vikings/warriors fighting an alien that looks like a wolf with tentacles, The difference between the phonemes /p/ and /b/ in Japanese. Insertion Sort | Insertion Sort Algorithm - Scaler Topics Below is simple insertion sort algorithm for linked list. We are only re-arranging the input array to achieve the desired output. How to earn money online as a Programmer? In this article, we have explored the time and space complexity of Insertion Sort along with two optimizations. In the extreme case, this variant works similar to merge sort. In 2006 Bender, Martin Farach-Colton, and Mosteiro published a new variant of insertion sort called library sort or gapped insertion sort that leaves a small number of unused spaces (i.e., "gaps") spread throughout the array. Analysis of insertion sort. So the sentences seemed all vague. a) insertion sort is stable and it sorts In-place That means suppose you have to sort the array elements in ascending order, but its elements are in descending order. Hence, The overall complexity remains O(n2). It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Reopened because the "duplicate" doesn't seem to mention number of comparisons or running time at all. Connect and share knowledge within a single location that is structured and easy to search. As the name suggests, it is based on "insertion" but how? This article introduces a straightforward algorithm, Insertion Sort. Worst, Average and Best Cases; Asymptotic Notations; Little o and little omega notations; Lower and Upper Bound Theory; Analysis of Loops; Solving Recurrences; Amortized Analysis; What does 'Space Complexity' mean ? Which of the following is not an exchange sort? For comparison-based sorting algorithms like insertion sort, usually we define comparisons to take, Good answer. In this worst case, it take n iterations of . Best-case : O (n)- Even if the array is sorted, the algorithm checks each adjacent . before 4. c) Insertion Sort To practice all areas of Data Structures & Algorithms, here is complete set of 1000+ Multiple Choice Questions and Answers. The best-case . Insertion sort is an in-place algorithm, meaning it requires no extra space. In the data realm, the structured organization of elements within a dataset enables the efficient traversing and quick lookup of specific elements or groups. Assignment 5 - The College of Engineering at the University of Utah In the context of sorting algorithms, Data Scientists come across data lakes and databases where traversing through elements to identify relationships is more efficient if the containing data is sorted. The worst case occurs when the array is sorted in reverse order. For example, centroid based algorithms are favorable for high-density datasets where clusters can be clearly defined. Conversely, a good data structure for fast insert at an arbitrary position is unlikely to support binary search. which when further simplified has dominating factor of n2 and gives T(n) = C * ( n 2) or O( n2 ), Let's assume that tj = (j-1)/2 to calculate the average case it is appropriate for data sets which are already partially sorted. By clicking Post Your Answer, you agree to our terms of service, privacy policy and cookie policy. We can optimize the swapping by using Doubly Linked list instead of array, that will improve the complexity of swapping from O(n) to O(1) as we can insert an element in a linked list by changing pointers (without shifting the rest of elements). Can anyone explain the average case in insertion sort? Pseudo-polynomial Algorithms; Polynomial Time Approximation Scheme; A Time Complexity Question; Searching Algorithms; Sorting . The inner while loop continues to move an element to the left as long as it is smaller than the element to its left. During each iteration, the first remaining element of the input is only compared with the right-most element of the sorted subsection of the array. Insertion sort is adaptive in nature, i.e. Bucket sort - Wikipedia communities including Stack Overflow, the largest, most trusted online community for developers learn, share their knowledge, and build their careers. Thus, the total number of comparisons = n*(n-1) ~ n 2 We can use binary search to reduce the number of comparisons in normal insertion sort. Now we analyze the best, worst and average case for Insertion Sort. Minimising the environmental effects of my dyson brain. The outer for loop continues iterating through the array until all elements are in their correct positions and the array is fully sorted. Best case: O(n) When we initiate insertion sort on an . K-Means, BIRCH and Mean Shift are all commonly used clustering algorithms, and by no means are Data Scientists possessing the knowledge to implement these algorithms from scratch. As stated, Running Time for any algorithm depends on the number of operations executed. This is mostly down to time and space complexity. We can reduce it to O(logi) by using binary search. How do I sort a list of dictionaries by a value of the dictionary? Direct link to ayush.goyal551's post can the best case be writ, Posted 7 years ago. Was working out the time complexity theoretically and i was breaking my head what Theta in the asymptotic notation actually quantifies. We have discussed a merge sort based algorithm to count inversions. Insertion Sort - GeeksforGeeks insertion sort keeps the processed elements sorted. Identifying library subroutines suitable for the dataset requires an understanding of various sorting algorithms preferred data structure types. b) Selection Sort Time Complexity of Quick sort. Fibonacci Heap Deletion, Extract min and Decrease key, Bell Numbers (Number of ways to Partition a Set), Tree Traversals (Inorder, Preorder and Postorder), merge sort based algorithm to count inversions. On the other hand, insertion sort is an . Theoretically Correct vs Practical Notation, Replacing broken pins/legs on a DIP IC package. No sure why following code does not work. You are confusing two different notions. Best case - The array is already sorted. c) Merge Sort What will be the worst case time complexity of insertion sort if the correct position for inserting element is calculated using binary search? The algorithm is based on one assumption that a single element is always sorted. If the cost of comparisons exceeds the cost of swaps, as is the case The algorithm starts with an initially empty (and therefore trivially sorted) list. At a macro level, applications built with efficient algorithms translate to simplicity introduced into our lives, such as navigation systems and search engines. So if the length of the list is 'N" it will just run through the whole list of length N and compare the left element with the right element. In this article, we have explored the time and space complexity of Insertion Sort along with two optimizations. Time complexity in each case can be described in the following table: Insertion Sort: Algorithm Analysis - DEV Community b) Statement 1 is true but statement 2 is false The selection of correct problem-specific algorithms and the capacity to troubleshoot algorithms are two of the most significant advantages of algorithm understanding. The selection sort and bubble sort performs the worst for this arrangement. For most distributions, the average case is going to be close to the average of the best- and worst-case - that is, (O + )/2 = O/2 + /2. The authors show that this sorting algorithm runs with high probability in O(nlogn) time.[9]. It is because the total time took also depends on some external factors like the compiler used, processors speed, etc. (n-1+1)((n-1)/2) is the sum of the series of numbers from 1 to n-1. Time complexity of insertion sort when there are O(n) inversions? O(n+k). Furthermore, algorithms that take 100s of lines to code and some logical deduction are reduced to simple method invocations due to abstraction. This gives insertion sort a quadratic running time (i.e., O(n2)). 8. What is the worst case example of selection sort and insertion - Quora Direct link to Cameron's post (n-1+1)((n-1)/2) is the s, Posted 2 years ago. which when further simplified has dominating factor of n2 and gives T(n) = C * ( n 2) or O( n2 ). For example, the array {1, 3, 2, 5} has one inversion (3, 2) and array {5, 4, 3} has inversions (5, 4), (5, 3) and (4, 3). , Posted 8 years ago. Direct link to Cameron's post Let's call The running ti, 1, comma, 2, comma, 3, comma, dots, comma, n, minus, 1, c, dot, 1, plus, c, dot, 2, plus, c, dot, 3, plus, \@cdots, c, dot, left parenthesis, n, minus, 1, right parenthesis, equals, c, dot, left parenthesis, 1, plus, 2, plus, 3, plus, \@cdots, plus, left parenthesis, n, minus, 1, right parenthesis, right parenthesis, c, dot, left parenthesis, n, minus, 1, plus, 1, right parenthesis, left parenthesis, left parenthesis, n, minus, 1, right parenthesis, slash, 2, right parenthesis, equals, c, n, squared, slash, 2, minus, c, n, slash, 2, \Theta, left parenthesis, n, squared, right parenthesis, c, dot, left parenthesis, n, minus, 1, right parenthesis, \Theta, left parenthesis, n, right parenthesis, 17, dot, c, dot, left parenthesis, n, minus, 1, right parenthesis, O, left parenthesis, n, squared, right parenthesis, I am not able to understand this situation- "say 17, from where it's supposed to be when sorted? Time and Space Complexities of all Sorting Algorithms - Interview Kickstart Intuitively, think of using Binary Search as a micro-optimization with Insertion Sort. But since the complexity to search remains O(n2) as we cannot use binary search in linked list. How to handle a hobby that makes income in US. . Sort array of objects by string property value, Sort (order) data frame rows by multiple columns, Easy interview question got harder: given numbers 1..100, find the missing number(s) given exactly k are missing, Image Processing: Algorithm Improvement for 'Coca-Cola Can' Recognition, Fastest way to sort 10 numbers? Thus, the total number of comparisons = n*(n-1) = n 2 In this case, the worst-case complexity will be O(n 2). If the inversion count is O(n), then the time complexity of insertion sort is O(n). With a worst-case complexity of O(n^2), bubble sort is very slow compared to other sorting algorithms like quicksort. In different scenarios, practitioners care about the worst-case, best-case, or average complexity of a function. The nature of simulating nature: A Q&A with IBM Quantum researcher Dr. Jamie We've added a "Necessary cookies only" option to the cookie consent popup. Find centralized, trusted content and collaborate around the technologies you use most. Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. Therefore overall time complexity of the insertion sort is O (n + f (n)) where f (n) is inversion count. Data Science and ML libraries and packages abstract the complexity of commonly used algorithms. How can I pair socks from a pile efficiently? the worst case is if you are already sorted for many sorting algorithms and it isn't funny at all, sometimes you are asked to sort user input which happens to already be sorted. Insertion sort algorithm involves the sorted list created based on an iterative comparison of each element in the list with its adjacent element. The list grows by one each time. However, insertion sort provides several advantages: When people manually sort cards in a bridge hand, most use a method that is similar to insertion sort.[2]. If the current element is less than any of the previously listed elements, it is moved one position to the left. The set of all worst case inputs consists of all arrays where each element is the smallest or second-smallest of the elements before it. Therefore overall time complexity of the insertion sort is O(n + f(n)) where f(n) is inversion count. We could see in the Pseudocode that there are precisely 7 operations under this algorithm. Binary Insertion Sort - Take this array => {4, 5 , 3 , 2, 1}. In contrast, density-based algorithms such as DBSCAN(Density-based spatial clustering of application with Noise) are preferred when dealing with a noisy dataset. If the value is greater than the current value, no modifications are made to the list; this is also the case if the adjacent value and the current value are the same numbers. Get this book -> Problems on Array: For Interviews and Competitive Programming, Reading time: 15 minutes | Coding time: 5 minutes. This doesnt relinquish the requirement for Data Scientists to study algorithm development and data structures. Please write comments if you find anything incorrect, or you want to share more information about the topic discussed above. Searching for the correct position of an element and Swapping are two main operations included in the Algorithm. Insertion Sort Algorithm in Java | Visualization and Examples Sorting is typically done in-place, by iterating up the array, growing the sorted list behind it. Where does this (supposedly) Gibson quote come from? When given a collection of pre-built algorithms to use, determining which algorithm is best for the situation requires understanding the fundamental algorithms in terms of parameters, performances, restrictions, and robustness. Insertion Sort algorithm follows incremental approach. In insertion sort, the average number of comparisons required to place the 7th element into its correct position is ____ The algorithm below uses a trailing pointer[10] for the insertion into the sorted list. Direct link to csalvi42's post why wont my code checkout, Posted 8 years ago. Worst Case Time Complexity of Insertion Sort. The number of swaps can be reduced by calculating the position of multiple elements before moving them. (numbers are 32 bit). Now inside the main loop , imagine we are at the 3rd element. Exhibits the worst case performance when the initial array is sorted in reverse order.b. While insertion sort is useful for many purposes, like with any algorithm, it has its best and worst cases. Efficient algorithms have saved companies millions of dollars and reduced memory and energy consumption when applied to large-scale computational tasks.