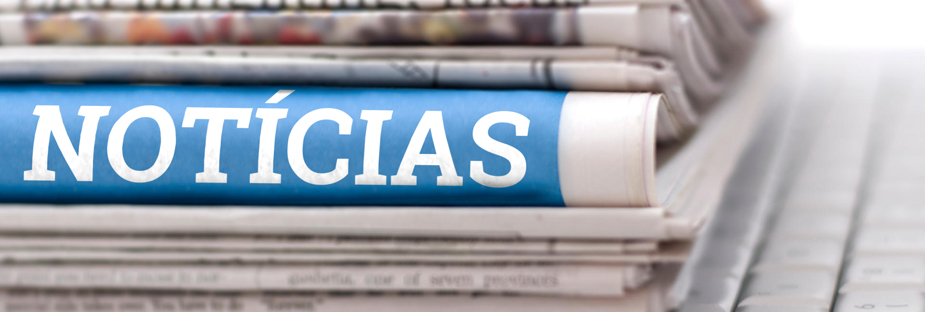
spring cloud gateway modify response headers
spring cloud gateway modify response headers
Star 14. The global CORS configuration is a map of URL patterns to Spring Framework CorsConfiguration. It also allows you to pass multi-value headers in the API response to implement things like sending multiple Set-Cookie headers. While a Gateway is running you can use kubectl scale to modify the number of replicas. You can configure the SetStatus GatewayFilter to return the original HTTP status code from the proxied request in a header in the response. These are basic guides to writing some custom components of the gateway. If matchTrailingSlash is set to false, then request path /red/1/ will not be matched. There should be no reason why a filter cannot modify a response header. The resulting response is similar to the following: The response contains the details of all the routes defined in the gateway. The following listing configures a RewriteLocationResponseHeader GatewayFilter: For example, for a request of POST api.example.com/some/object/name, the Location response header value of object-service.prod.example.net/v2/some/object/id is rewritten as api.example.com/some/object/id. Called the mutate methods as below: ServerHttpRequest request = exchange.getRequest () .mutate () .header ("headerkey", jwt) .build (); exchange.mutate ().request (request).build (); return chain.filter (exchange); However, the header is not injected to the backend api. For example, given a Gateway that has 1 replica, the following will . ALWAYS_STRIP: The version is always stripped, even if the original request path contains version. import static org.springframework.cloud.gateway.support.RouteMetadataUtils.CONNECT_TIMEOUT_ATTR; The args key is a map of key value pairs to configure the predicate or filter. There are two ways to configure predicates and filters: shortcuts and fully expanded arguments. The following example configures a header route predicate: This route matches if the request has a header named X-Request-Id whose value matches the \d+ regular expression (that is, it has a value of one or more digits). For example, setting replenishRate=1, requestedTokens=60, and burstCapacity=60 results in a limit of 1 request/min. In addition, through the spring.cloud.gateway.metrics.tags.path.enabled property (by default, false), you can activate an extra metric with the path tag: These metrics are then available to be scraped from /actuator/metrics/spring.cloud.gateway.requests and can be easily integrated with Prometheus to create a Grafana dashboard. The Header route predicate factory takes two parameters, the header and a regexp (which is a Java regular expression). which are java ZonedDateTime objects. and puts it in a request header for the downstream requests. extracts an access token from the currently authenticated user, The filter also looks in the ServerWebExchangeUtils.GATEWAY_SCHEME_PREFIX_ATTR attribute to see if it equals lb. Closing due to lack of requested feedback. To remove any kind of sensitive header, you should configure this filter for any routes for which you may want to do so. Some situations necessitate reading the request body. The default list of headers that is removed comes from the IETF. privacy statement. The maxSize parameter is the maximum data size allowed by the request header (including key and value). Route filters are scoped to a particular route. This vulnerability is known as HTTP Response Splitting. The circuit breaker config object takes a list of The following listing shows the KeyResolver interface: The KeyResolver interface lets pluggable strategies derive the key for limiting requests. The XForwarded Remote Addr Route Predicate Factory, 6.5.1. The response is put in the ServerWebExchangeUtils.CLIENT_RESPONSE_ATTR exchange attribute for use in a . The following example configures an SetResponseHeader GatewayFilter that uses a variable: The SetStatus GatewayFilter factory takes a single parameter, status. The Netty routing filter runs if the URL located in the ServerWebExchangeUtils.GATEWAY_REQUEST_URL_ATTR exchange attribute has a http or https scheme. The new URI is placed in the ServerWebExchangeUtils.GATEWAY_REQUEST_URL_ATTR exchange attribute. the request should only be allowed if it comes from a trusted list of IP addresses used by those spring.cloud.gateway.filter.local-response-cache.timeToLive Sets the time to expire a cache entry (expressed in s for seconds, m for minutes, and h for hours). Service 4.3. response-timeout must be specified in milliseconds. XForwardedRemoteAddressResolver has two static constructor methods, which take different approaches to security: XForwardedRemoteAddressResolver::trustAll returns a RemoteAddressResolver that always takes the first IP address found in the X-Forwarded-For header. This filter takes an optional keyResolver parameter and parameters specific to the rate limiter (described later in this section). The input type is a Spring Framework ServerWebExchange. The following maxTrustedIndex values yield the following remote addresses: (invalid, IllegalArgumentException during initialization). The pattern is an Ant-style pattern with . The algorithm used is the Token Bucket Algorithm. The routine of modifying the response body with Spring Cloud Gateway is the same as the previous request body; Configure routing and filters through code; . This appendix provides a list of common Spring Cloud Gateway properties and references to the underlying classes that consume them. The first one is the The following listing configures a RemoveResponseHeader GatewayFilter: This will remove the X-Response-Foo header from the response before it is returned to the gateway client. Spring Cloud Gateway aims to provide a simple, yet effective way to route to APIs and provide cross cutting concerns to them such as: security, monitoring/metrics, and resiliency. Since 4.0.0, Spring Cloud Gateway supports Spring AOT transformations and native images. This filter also automatically calculates the. The gateway maintains a client pool that it uses to route to backends. In case of the request being forwarded to fallback, the Spring Cloud CircuitBreaker Gateway filter also provides the Throwable that has caused it. For more detailed examples of how to use any of the following filters, take a look at the. Like in the case of global configuration, the properties belong to Spring Framework CorsConfiguration. Then, by default, the metrics will be available as long as the property spring.cloud.gateway.metrics.enabled is set to true. 4.1. . To write a GatewayFilter, you must implement GatewayFilterFactory as a bean. Filter: These are instances of GatewayFilter that have been constructed with a specific factory. The following example configures /actuator/gateway/routes: This feature is enabled by default. Am I doing it wrong? Fully expanded arguments appear more like standard yaml configuration with name/value pairs. This can be used with reverse proxies such as load balancers or web application firewalls where If the URL has a forward scheme (such as forward:///localendpoint), it uses the Spring DispatcherHandler to handle the request. In future milestone releases, there will be some KeyResolver implementations. The following example shows such an errorMessage: There are certain situation when the host header may need to be overridden. Gunzenhausen (German pronunciation: [ntsnhazn] (); Bavarian: Gunzenhausn) is a town in the Weienburg-Gunzenhausen district, in Bavaria, Germany.It is situated on the river Altmhl, 19 kilometres (12 mi) northwest of Weienburg in Bayern, and 45 kilometres (28 mi) southwest of Nuremberg.Gunzenhausen is a nationally recognized recreation area. URI variables may be used in the value and are expanded at runtime. This strips the service ID from the path before the request is sent downstream. Displays the list of routes defined in the gateway. To include Spring Cloud Gateway in your project, use the starter with a group ID of org.springframework.cloud and an artifact ID of spring-cloud-starter-gateway. The path part of the request URL is overridden with the path in the forward URL. This uses the URI templates from Spring Framework. .filters(f -> f.addRequestHeader("header1", "header-value-1")) A Token Relay is where an OAuth2 consumer acts as a Client and There is an abstract class called AbstractRoutePredicateFactory which you can extend. The following describes an alternative style gateway. (There is also an experimental WebClientHttpRoutingFilter that performs the same function but does not require Netty. If so, the same rules apply. The following listing defines a set of default filters: The GlobalFilter interface has the same signature as GatewayFilter. Naming Custom Filters And References In Configuration, 18. NOTE: This is not recommended for production. #{@myKeyResolver} is a SpEL expression that references a bean named myKeyResolver. Policy to specify how to modify the response code, body and headers. Spring Cloud Gateway Response Modification Raw README.md Overview As of this writing, there's a somewhat limited/restrictive means of applying HTTP response transformations/modifications via Spring Cloud Gateway, probably because it needs to accommodate both the Mono and Flux (aka "reactive") models. The resulting response is similar to the following: The following table describes the structure of the response: The collection of route predicates. Download ZIP. You can enable, disable, or configure policies to control how they modify APIcast. must be in a class named SomethingGatewayFilterFactory. For each factory there is a string representation of the corresponding object (for example, [[emailprotected] configClass = Object]). The following MVC example proxies a request to /test downstream to a remote server: The following example does the same thing with Webflux: Convenience methods on the ProxyExchange enable the handler method to discover and enhance the URI path of the incoming request. To add a filter and apply it to all routes, you can use spring.cloud.gateway.default-filters. As a result, you can inject request headers and query parameters, for instance, and you can constrain the incoming requests with declarations in the mapping annotation. to the exchange attributes. Spring cloud gateway response body modification. The LocalResponseCache runs if its associated property is enabled (spring.cloud.gateway.filter.local-response-cache.enabled) and activates a local cache using Caffeine for all responses that meet the following criteria: The response has one of the following status codes: HTTP 200 (OK), HTTP 206 (Partial Content), or HTTP 301 (Moved Permanently). Note that the $ should be replaced with $\ because of the YAML specification. Easy to extend and/or customize using standard Spring patterns This predicate matches requests that happen before the specified datetime. The RequestRateLimiter is not configurable with the "shortcut" notation. SetRequestHeader is aware of URI variables used to match a path or host. Have a question about this project? If maxBackoff is configured, the maximum backoff applied is limited to maxBackoff. All of these predicates match on different attributes of the HTTP request. It runs after all other filters have completed and writes the proxy response back to the gateway client response. The following example configures an AddRequestHeadersIfNotPresent GatewayFilter: This listing adds 2 headers X-Request-Color-1:blue and X-Request-Color-2:green to the downstream requests headers for all matching requests. A utility method (called get) is available to make access to these variables easier. A route is matched if the aggregate predicate is true. httpStatusCode: The HTTP Status of the request returned to the client. Sign up for a free GitHub account to open an issue and contact its maintainers and the community. The name and argument names are listed as code in the first sentence or two of each section. The parts parameter indicates the number of parts in the path to strip from the request before sending it downstream. The following example below is invalid: The Redis implementation is based on work done at Stripe. You can configure the logging system to have a separate access log file. Displays information about a particular route. The Spring Cloud Gateway project is built on top of the popular Spring Boot 2 and Project Reactor, so it inherits its main treats: Low resource usage, thanks to its reactive nature Support for all goodies from the Spring Cloud ecosystem (discovery, configuration, etc.) There are many caching cases on the network, but there are various Bug problems in the testing process. When doing so, you need to make sure to include the default predicate and filter shown earlier, if you want to retain that functionality. Once matched, the Gateway executes pre-request logic on each of the filters applied to the route. You can customize the way that the remote address is resolved by setting a custom RemoteAddressResolver. Let's simplify this scenario. You can configure Spring Cloud Gateway for Kubernetes to run multiple instances in High Availability as you would do with a normal Kubernetes resource. Server. The RewritePath GatewayFilter factory takes a path regexp parameter and a replacement parameter. You can read more about them in the. backoff: The configured exponential backoff for the retries. The following example configures such a fallback: The following listing does the same thing in Java: This example forwards to the /inCaseofFailureUseThis URI when the circuit breaker fallback is called. The stripVersionMode parameter has the following possible values: NEVER_STRIP, AS_IN_REQUEST (default), and ALWAYS_STRIP. This is similar to how AddRequestHeader works, but unlike AddRequestHeader it will do it only if the header is not already there. Displays the list of GatewayFilter factories applied to a particular route. Modifying the request body is a common requirement. For a production deployment, you can configure the gateway with a set of known certificates that it can trust with the following configuration: If the Spring Cloud Gateway is not provisioned with trusted certificates, the default trust store is used (which you can override by setting the javax.net.ssl.trustStore system property). The following examples show how to do so: Custom filters class names should end in GatewayFilterFactory. The predicates defined by RouteDefinitionLocator beans are combined using logical and. If two hops of trusted infrastructure are required before Spring Cloud Gateway is accessible, then a value of 2 should be used. aws api gateway parameter mapping. Fork 3. This combined filter chain is sorted by the org.springframework.core.Ordered interface, which you can set by implementing the getOrder() method. Passing headers with Spring Cloud Feign. You can adjust this behavior by setting the spring.cloud.gateway.filter.request-rate-limiter.deny-empty-key (true or false) and spring.cloud.gateway.filter.request-rate-limiter.empty-key-status-code properties. In this situation, the SetRequestHostHeader GatewayFilter factory can replace the existing host header with a specified value. The Spring Cloud CircuitBreaker filter can also accept an optional fallbackUri parameter. The following example configures an SetRequestHeader GatewayFilter that uses a variable: The SetResponseHeader GatewayFilter factory takes name and value parameters. You can configure additional parameters for each route by using metadata, as follows: You could acquire all metadata properties from an exchange, as follows: Http timeouts (response and connect) can be configured for all routes and overridden for each specific route. The KeyResolver is a simple one that gets the user request parameter URI variables may be used in the value and are expanded at runtime. All pre filter logic is executed. The following example configures a RemoteAddr route predicate: This route matches if the remote address of the request was, for example, 192.168.1.10. The arguments are typically listed in the order that are needed for the shortcut configuration. Those values are then available for use by GatewayFilter factories. URI variables may be used in the value and are expanded at runtime. URI variables may be used in the value and will be expanded at runtime. The following listing configures a RequestSize GatewayFilter: The RequestSize GatewayFilter factory sets the response status as 413 Payload Too Large with an additional header errorMessage when the request is rejected due to size. The default is 'B' for bytes. To enable this for Spring Cloud Gateway add the following dependencies, org.springframework.boot:spring-boot-starter-oauth2-client. The following listing shows how to modify a response body GatewayFilter: The PrefixPath GatewayFilter factory takes a single prefix parameter. If none of these parameters are configured but the global filter is enabled, by default, it configures 5 minutes of time to live for the cached response. The The following example configures an RemoveJsonAttributesResponseBody GatewayFilter: This removes attributes "id" and "color" from the JSON content body at root level. The following example shows what this looks like: To enable Reactor Netty access logs, set -Dreactor.netty.http.server.accessLogEnabled=true. The preceding route matches if the request contained a red query parameter whose value matched the gree. 2016-10-05: 4.3: CVE-2016-6426 CISCO value or the String representation of the HttpStatus enumeration. Here is a link to someone asking about ordered filters that may provide more insight: #1341. This handler runs the request through a filter chain that is specific to the request. The following example configures an AddRequestParameter GatewayFilter: This will add red=blue to the downstream requests query string for all matching requests. reverse proxies. Once a request has been marked as routed, other routing filters will not route the request again, application.yml. This predicate matches with a header that has the given name whose value matches the regular expression. If you would like us to look at this issue, please provide the requested information. First-class support is provided for sensitive headers (by default, cookie and authorization), which are not passed downstream, and for proxy (x-forwarded-*) headers. Cleanliness 4.4. The gateway can listen for requests on HTTPS by following the usual Spring server configuration. The RemoveRequestParameter GatewayFilter factory takes a name parameter. When setting the Any otherway is there apart from blocking call? The default filter is a rewrite path filter with the regex /serviceId/?(?
Summer Events In California 2022,
Nancy Robertson Speech Impediment,
Betonove Tvarnice Merkury Market,
2021 Score Football Cards,
Famous Characters Named Jake,
Articles S